I just got the picture from someone that killed one of these resistors yesterday while working on a dc/dc converter for a solar to battery storage / grid solution.
Just an example to show that its not that uncommon 
Everybody needs to start somewhere.
I am also no software developer. So i imagine my code would be scary to everyone doing serious stuff.
But thats a simple PID loop for a fogger i build:
void PIDpump(){ //this gets called by ADC_measure which gets called by timer. Repeatable intervall for PID loop
//BackEMF -> value of motor speed 0-900
//requestedFog -> 0-700 needed motor speed.
//OCR1B -> output stuff 0-2500 "pwm value" lowest value 250, highest value 2075
if(requestedFog >= 1){ // we need to start fogging, calculate PID
error = requestedFog - BackEMF; //calculate velocity error term reqFog is set point while BackEMF is current value
cumError += error; //I term, gets integrated by adding
if(cumError >= 500){ // wind up correction
cumError = 500; // I term, set to min
}
if(cumError <= -500){ // wind up correction
cumError = -500; // I term, set to min
}
outputValue = (Kp * error) + (Ki * cumError) + (Kd * (error - lastError)); //calculate output
if(outputValue > 2075){ // limit output to max and minimum PWM value
outputValue = 2075;
}else if(outputValue < 270){
outputValue = 270;
}
lastError = error; // safe last error value for D calculation
OCR1B = outputValue; // write PWM value to register
}else{ //we dont need to make fog -> stop PID and reset
OCR1B = 0; //write 0 to PWM register
error = 0; //error 0
lastError = 0; //last error 0
cumError = 0; //integrated error 0
}
}
The real PID stuff is essentially just these lines:
error = requestedFog - BackEMF; //calculate velocity error term reqFog is set point while BackEMF is current value
cumError += error; //I term, gets integrated by adding
outputValue = (Kp * error) + (Ki * cumError) + (Kd * (error - lastError)); //calculate output
lastError = error; // safe last error value for D calculation
First the error gets calculated by just getting the difference between set point and current value.
This error term gets added each time the PID loop runs. Thats for the I term. We essentially integrate the error over time.
The output value gets calculate by just adding all the PID components.
Lastly the current error gets saved to calculate the D term at the next run.
Very simple, so no real need for a library.
My loop includes switching the PID off and having wind up correction for the integrated error, otherwise high integrated values would lead to oscillations.
Softstart could be implemented by increasing the PWM duty at startup from 0 to the PID output value slowly.
I would also limit the maximum duty-cycle to make the tec always run with its optimum COP.
No need to supply it 12V if it will have enough power at 7V.
Running it at 12V at startup would increase losses and make a bigger power supply necessary.
Stepdown:
I thought i read somewhere that you used a 10uH inductor, my bad.
Your assumption of controlling the voltage by duty cycle is correct if your inductor is big enough to have no zero current times. With 50uH and your load, that works out perfectly.
The circuit is actually essentially a stepdown regulator with common positive. The simulation i posted is the same but common negative.
That circuit is nice because you can get away with a n-ch fet (better parameters) and can use a simple ground related control signal (0-5V for example) but pay for it by having no ground reference on your load. But thats completely fine here.
YES! Sorry! Completely true, i messed up and somehow thought about step up which essentially shorts the power supply with the fet.
You just need to make sure that your fet can handle the max current and max voltage and max power dissipation. It also needs to be completely on. That could be a problem here.
U and I should be clear, your fet is rated for more then enough. But your nano will only output 5V to control your fet. Your fet is barely on enough. You will get around 40mR at 5V instead of the 5mR at 10V.
So your efficiency will not be great, at 10A you are looking at around 4W of losses.
Not great not terrible.
Your diode needs to be fast. You will get losses because of the higher forward voltage. The diode takes the current while the fet isnt, so it needs to carry the full load current.
Because you got a much bigger inductor, your current never gets to zero. Therefore the load current is always shared by the capacitor and inductor. You will not need a 6A capable capacitor. Around 0.75A RMS should be enough.
You load would still receive around 100mV of ripple which is quite much for a power supply but it should be ok for a tec.
But you also need an input cap. The peak current to “charge” the inductor needs to be supplied fast, caps will do that.
I just borrowed a picture: (common ground - q1 would be your fet with q2 being the diode)
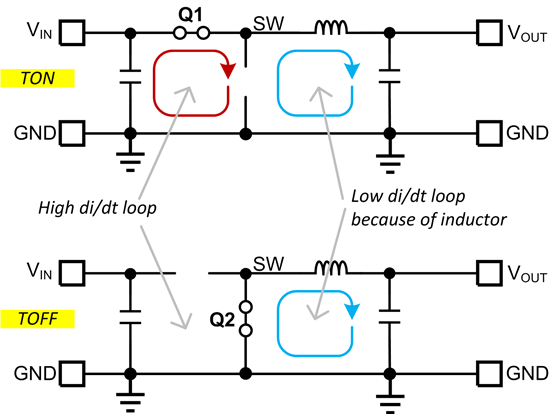
The blue and the red loop needs to be small otherwise noise will be radiated.
If you got no input capacitor, the high di/dt loop will be huge, extending to your power supply.
You need the input cap to keep the loop small and supply the high peak currents with local capacitance.